変数の定義
const hoge = document.querySelector('.hoge');
let | var | const | |
---|---|---|---|
再宣言 | × | ◯ | × |
再代入 | ◯ | ◯ | × |
繰り返し構文 | ◯ | ◯ | × |
スコープ | ブロック | 関数 | ブロック |
- 基本はconstで定義
- 繰り返し構文のみletを定義
- var万能だがエラーにつながる可能性が高い
Ready関数
document.addEventListener('DOMContentLoaded', function() {
});
要素の取得
HTML
document.documentElement;
Body
document.body;
タグ
// querySelector
document.querySelector('div');
// getElement
document.getElementsByTagName('div');
ID
// querySelector
document.querySelector('#id');
// getElement
document.getElementById('id');
クラス
// querySelector
document.querySelector('.class');
// getElement
ocument.getElementsByClassName('class');
※querySelectorは最初の要素を返す
複数の要素
document.querySelectorAll('.hoge');
document.querySelectorAll('.hoge, .fuga');
子孫要素
const parent = document.querySelector('.parent');
parent.querySelectorAll('.child');
//もしくは
const parent = document.getElementsByClassName('parent');
parent.getElementsByClassName('child');
全ての子要素
document.querySelector('.parent').children;
特定の子要素
const parent = document.querySelector('.parent');
parent.querySelector('.child');
親要素
document.querySelector('.hoge').parentNode;
先祖要素
const hoge = document.querySelector('.hoge');
hoge.closest('.fuga');
直前の兄弟要素
document.querySelector('.hoge').previousElementSibling;
直後の兄弟要素
document.querySelector('.hoge').nextElementSibling;
全ての兄弟要素
const parent = document.querySelector('.hoge').parentNode;
parent.children;
特定の兄弟要素
hoge.parentElement.querySelector('.fuga');
存在確認
要素の存在確認
//単一要素
const hoge = document.querySelector('.hoge');
if (hoge !== null) {
}
//複数要素
const hoge = document.querySelectorAll('.hoge');
if (hoge.length > 0) {
}
クラスの存在確認
if (hoge.classList.contains('fuga')) {
}
クラスの操作
クラスの追加
hoge.classList.add('class');
hoge.classList.add('class1', 'class2');
クラスの削除
hoge.classList.remove('class');
hoge.classList.remove('class1', 'class2');
クラスのトグル操作
hoge.classList.toggle('class');
クラスの取得
document.querySelector('.hoge').className;
複数要素の処理
for
const hoges = document.querySelectorAll('.hoge');
for (let i = 0; i < hoges.length; i++) {
hoges[i].classList.add('class');
}
for await...of
const hoges = document.querySelectorAll('.hoge');
for await (let hoge in hoges) {
hoge.classList.add('class');
}
for...in
const hoges = document.querySelectorAll('.hoge');
for (let hoge in hoges) {
hoge.classList.add('class');
}
for...of
const hoges = document.querySelectorAll('.hoge');
for (let hoge of hoges) {
hoge.classList.add('class');
}
forEach
const hoges = document.querySelectorAll('.hoge');
hoges.forEach((hoge) => {
hoge.classList.add('class');
});
forEach(ナンバリング)
const hoges = document.querySelector('.hoge');
const lists = hoges.querySelectorAll('li');
lists.forEach((list, index) => {
let number = index + 1;
list.setAttribute('data-number', number);
});
/*
<ul class="hoge">
<li data-number="1"></li>
<li data-number="2"></li>
<li data-number="3"></li>
</ul>
*/
forEach(最初と最後を操作)
const hoges = document.querySelector('.hoge');
const lists = hoges.querySelectorAll('li');
lists.forEach((list, index, array) => {
if (index === 0) {
list.classList.add('first');
}
if (index === array.length - 1) {
list.classList.add('last');
}
});
/*
<ul class="hoge">
<li class="first"></li>
<li></li>
<li class="last"></li>
</ul>
*/
異なる要素の場合
const selectors = document.querySelectorAll('.hoge, .fuga');
selectors.forEach(selector => {
selector.setAttribute('data-hoge', 'ほげ');
});
//または
const selectors = ['.hoge', '.fuga'];
selectors.forEach(selector => {
let hoge = document.querySelector(selector);
hoge.setAttribute('data-hoge', 'ほげ');
}):
属性値の操作
属性値の取得
hoge.getAttribute('data-hoge');
属性値のセット
hoge.setAttribute('data-hoge', 'fuga');
属性値の削除
hoge.removeAttribute('data-hoge');
スタイルの操作
スタイルの取得
hoge.style.backgroundColor;
スタイルのセット
hoge.style.backgroundColor = '#000';
スタイルの複数セット
hoge.style.color = '#fff';
hoge.style.backgroundColor = '#000';
プロパティ名の書き方
//color
color
//background-color
backgroundColor
//-webkit-transform
webkitTransform
要素の操作
要素のタグ名を取得
document.querySelector('.hoge').tagName;
paddingを含む要素のサイズ取得
//高さ
document.querySelector('.hoge').clientHeight;
//横幅
document.querySelector('.hoge').clientWidth;
paddingとborderを含む要素のサイズ取得
//高さ
document.querySelector('.hoge').offsetHeight;
//横幅
document.querySelector('.hoge').offsetWidth;
要素の座標取得
const rect = document.querySelector('.hoge').getBoundingClientRect();
//ページ上部から要素上部
rect.top;
//ページ左端から要素右部
rect.right;
//ページ上部から要素下部
rect.bottom;
//ページ左端から要素左部
rect.left;
要素内のテキスト操作
//取得
document.querySelector('.hoge').textContent;
//セット
document.querySelector('.hoge').textContent = 'ほげ';
要素内のHTML操作
//取得
document.querySelector('.hoge').innerHTML;
//セット
document.querySelector('.hoge').innerHTML = '<span>ほげ</span>';
要素の生成
const hoge = document.createElement('div');
div.className = 'hoge'
div.innerHTML = '<span>ほげ</span>'
/*
<div class="hoge">
<span>ほげ</span>
</div>
*/
要素の削除
const hoge = document.querySelector('.hoge');
hoge.remove(); //「hoge」クラスを持ったエレメントを削除
要素の置き換え
const hoge = document.querySelector('.hoge');
const fuga = document.createElement('div');
fuga.className = 'fuga'
fuga.innerHTML = '<span>ふが</span>'
hoge.parentNode.replaceChild(fuga, hoge);
/*
<div class="fuga">
<span>ふが</span>
</div>
*/
要素の前に挿入
const hoge = document.querySelector('.hoge');
const fuga = document.createElement('div');
fuga.className = 'fuga'
fuga.innerHTML = '<span>ふが</span>'
hoge.parentNode.insertBefore(fuga, hoge);
/*
<div class="fuga">
<span>ふが</span>
</div>
<div class="hoge">
<span>ほげ</span>
</div>
*/
要素の後に挿入
const hoge = document.querySelector('.hoge');
const fuga = document.createElement('div');
fuga.className = 'fuga'
fuga.innerHTML = '<span>ふが</span>'
hoge.parentNode.insertBefore(fuga, hoge.nextElementSibling);
/*
<div class="hoge">
<span>ほげ</span>
</div>
<div class="fuga">
<span>ふが</span>
</div>
*/
要素の先頭に挿入
const hoge = document.querySelector('.hoge');
const fuga = document.createElement('div');
fuga.className = 'fuga'
fuga.innerHTML = '<span>ふが</span>'
hoge.insertBefore(fuga, hoge.firstElementChild);
要素の最後に挿入
const hoge = document.querySelector('.hoge');
const fuga = document.createElement('div');
fuga.className = 'fuga'
fuga.innerHTML = 'ふが'
hoge.appendChild(fuga);
要素を囲う
const hoge = document.querySelector('.hoge');
hoge.outerHTML = `<div class="fuga">${hoge.outerHTML}</div>`;
/*
<div class="fuga">
<div class="hoge">
<span>ほげ</span>
</div>
</div>
*/
要素の中身を囲う
const hoge = document.querySelector('.hoge');
hoge.innerHTML = `<div class="fuga">${hoge.innerHTML}</div>`;
/*
<div class="hoge">
<div class="fuga">
<span>ほげ</span>
</div>
</div>
*/
要素の表示・非表示
//表示
document.querySelector('.hoge').style.display = 'block';
//非表示
document.querySelector('.hoge').style.display = 'none';
要素のフェードイン・アウト
//フェードイン
const hoge = document.querySelector('.hoge');
hoge.style.display = 'none';
hoge.style.opacity = 0;
document.querySelector('.button').addEventListener("click", function () {
hoge.style.display = 'block';
hoge.animate(
[
{ opacity: 0 },
{ opacity: 1 },
],
{
duration: 1000,
easing: "linear",
fill: "forwards",
}
)
});
//フェードアウト
const hoge = document.querySelector('.hoge');
document.querySelector('.button').addEventListener("click", function () {
setTimeout(() => {
hoge.style.display = 'none';
}, 1000);
hoge.animate(
[
{ opacity: 1 },
{ opacity: 0 },
],
{
duration: 1000,
easing: "linear",
fill: "forwards",
}
)
});
※animateのスタイルでスライドイン・アウトやスケールも可。display操作はなくてもよい。
クラス付与でCSSアニメーションを使ったほうが楽かも。
イベントの操作
ロードイベント
window.addEventListener('load', function(){
});
クリックイベント
const hoge = document.querySelector('.hoge');
hoge.addEventListener('click', function(){
});
スクロールイベント
window.addEventListener('scroll', function(){
if (window.scrollY > 200) { //200pxスクロールで発火
//発火イベント
}
});
フォームの操作
送信イベント
const form = document.querySelector('#hoge');
form.addEventListener('submit', function(){
});
テキスト
const input = document.querySelector('input');
input.addEventListener('input', function () {
if(input.value !== '') {
document.querySelector('.hoge').textContent = 'ほげ';
}
});
※'change'でも可能だが、テキストボックスのフォーカスを外す必要があるので'input'を推奨
チェックボックス
checkbox.addEventListener('change', function(){
if(checkbox.checked) {
button.disabled = false;
} else {
button.disabled = true;
}
});
ラジオボタン
const radioButtons = document.querySelectorAll('input[type="radio"][name="fuga"]');
radioButtons.forEach((radio) => {
radio.addEventListener('change', function(){
document.querySelector('.hoge').textContent = radio.value;
});
});
セレクトボックス
const select = document.querySelector('#select');
select.addEventListener('change', function(){
document.querySelector('.hoge').textContent = select.value;
});
テキストの操作
数値をカンマ区切りに
const number = 1000000;
number.toLocaleString();
//1,000,000
文字列の置換
const hoge = 'ほげ';
hoge.replace('ほげ', 'ふが');
//ふが
文字列の削除
const hoge = 'ほげふが';
hoge.replace('ほげ', '');
//ふが
文字列の分割
const weeks = '日,月,火,水,木,金,土';
const week = weeks.split(',');
//["日","月","火","水","木","金","土"]
week[0] //日
week[5] //木
テキストの切り出し
const hoge = 'ほげふが';
hoge = hoge.substring(2);
//ふが
hoge = hoge.substring(2,3);
//げふ
条件分岐
if文
if (hoge === 0) {
fuga.clasList.add('is-show');
}
else文
if (hoge === 0) {
fuga.clasList.add('is-show');
} else {
fuga.clasList.remove('is-show');
}
else if文
if (hoge === 0) {
fuga.clasList.add('is-show');
} else if(hoge > 0) {
fuga.clasList.remove('is-show');
}
switch文
switch (hoge) {
case 0:
fuga.clasList.add('is-show');
break;
default:
fuga.clasList.remove('is-show');
break;
}
三項演算子
hoge === 0 ? fuga.clasList.add('is-show') : fuga.clasList.remove('is-show');
比較演算子の種類
== //等価演算子(値を比較)
=== //厳密等価演算子(値と型を比較)
> | 左辺が右辺よりも大きい場合はtrue |
>= | 左辺が右辺よりも大きいか等しい場合はtrue |
< | 左辺が右辺よりも小さい場合はtrue |
<= | 左辺が右辺よりも小さいか等しい場合はtrue |
=== (==) | 左辺と右辺が等しい場合はtrue |
!== (!=) | 左辺と右辺が等しくない場合はtrue |
文字列の部分一致
if (hoge.match('ほげ')) {
hoge.clasList.add('fuga');
}
ブラウザ操作
セッションストレージ
ブラウザを閉じると消える
//セット
sessionStorage.setItem('key', 'value');
//取得
sessionStorage.getItem('key');
//削除
sessionStorage.removeItem('key');
//全データ削除
sessionStorage.clear();
ローカルストレージ
消えない
//セット
localStorage.setItem('key', 'value');
//取得
localStorage.getItem('key');
//削除
localStorage.removeItem('key');
//全データ削除
localStorage.clear();
クッキー
有効期限を持たせられる
//読み込み
document.cookie;
//書き込み
document.cookie = "key=value";
//有効期限
document.cookie = "key=value; max-age=◯◯"; //◯◯秒後
document.cookie = "key=value; expires=Mon, 31 Aug 2020 15:00:00 GMT"; //日付指定
//削除
document.cookie = "key=value; max-age=0";
テンプレートリテラル
const money = '<span>' + price + '円</span>';
↓
const money = `<span>${price}円</span>`;
よく使うJS
Fetch API
//カレンダーを日本の祝日に対応させる一例
const holidays_jp = 'https://holidays-jp.github.io/api/v1/date.json';
fetch(holidays_jp)
.then((response) => response.json())
.then((holidaysData) => {
const holidays = Object.keys(holidaysData);
holidays.forEach((holiday) => {
const targets = document.querySelectorAll(`[data-date="${holiday}"]`);
targets.forEach((target) => {
target.classList.add('holiday');
});
});
});
Function
//共通の処理は関数にまとめると便利
const showButton = document.querySelector('.show-button');
const closeButton = document.querySelector('.close-button');
const cancelButton = document.querySelector('.cancel-button');
const target = document.querySelector('.target');
showButton.addEventListener('click', showTarget);
closeButton.addEventListener('click', closeTarget);
cancelButton.addEventListener('click', closeTarget);
function showTarget() {
target.classList.add('is-show');
}
function closeTarget() {
target.classList.remove('is-show');
}
setTimeout
setTimeout(() => {
hoge.close();
}, 500);//ミリ秒
モーダル操作
hoge.showModal();//開く
hoge.close();//閉じる
※<dialog>
タグを使う際に使用。
クリップボードにコピー
//<button type="button" class="copy-button" data-code="hoge">コピー</button>
const copyButton = document.querySelector('.copy-button');
copyButton.addEventListener('click', function () {
const element = document.createElement('input');
element.value = this.getAttribute('data-code');
document.body.appendChild(element);
element.select();
document.execCommand('copy');
document.body.removeChild(element);
alert('コピーしました');
});
この記事を書いた人
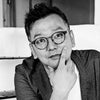
モリタオウ
株式会社テンカ 代表取締役 / ウェブクリエイター / グラフィックデザイナー
1977年12月20日生まれ。広告代理店や企業広報を経て、2007年12月にデザイン事務所「モリタ・クリエイト」を創業。2022年12月に「株式会社テンカ」を設立。